libft: reinventing the wheel?
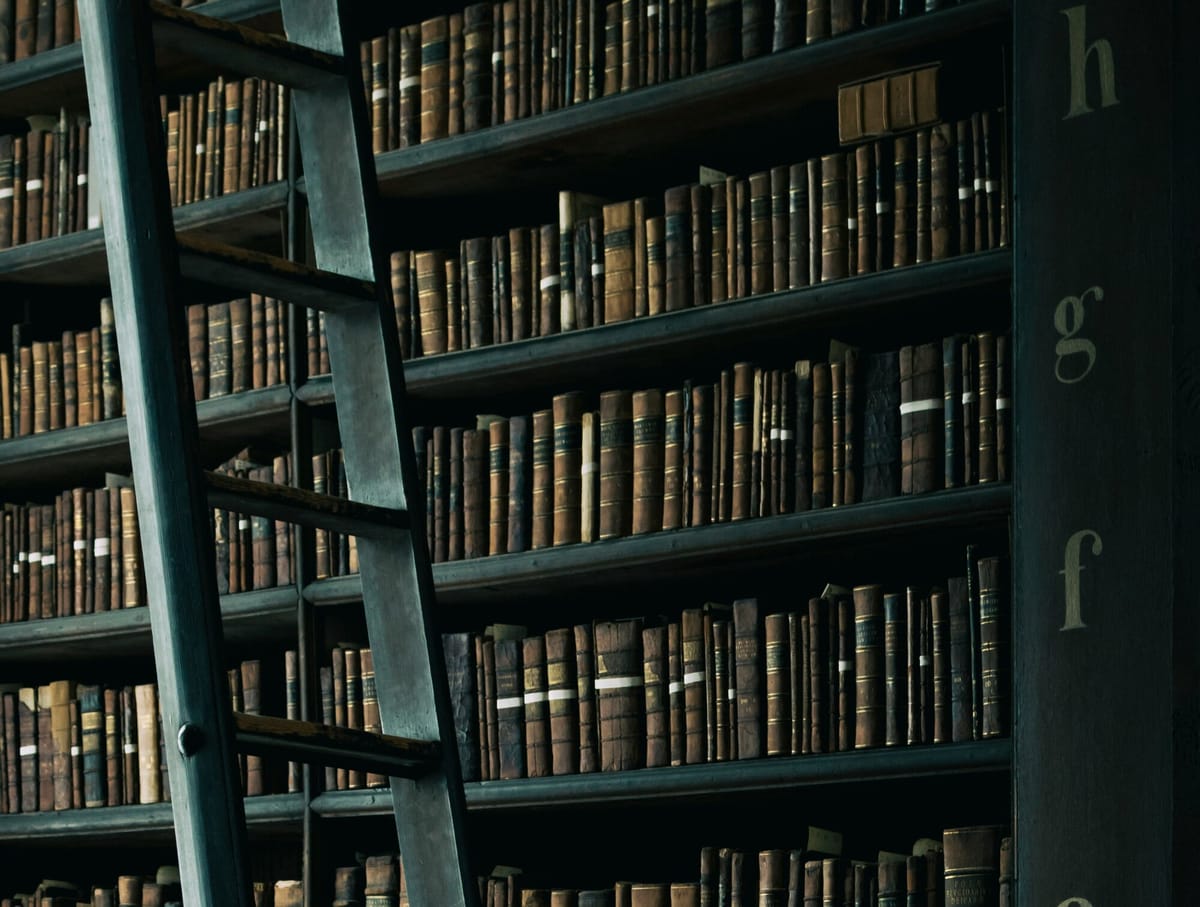
So I'm still in Brazil applying for my visa, but I can already start developing my projects from 42 so let's talk a little about the first project.
It's very common for us to use the standard C library functions like printf
, atoi
, malloc
, strdup
, and many others. However, most people never stop to think about how these functions actually work, the code behind them that we never see.
For this first project, I had to rewrite a set of functions from the libc
. They have the same prototypes and implement the same behaviors as the originals. In future projects, I will only be allowed to use these functions I wrote and not the original ones.
Here are the functions I recreated:
// Functions from libc
isalpha() strlcpy()
isdigit() strlcat()
isalnum() strlen()
isascii() strchr()
isprint() strrchr()
memset() strncmp()
memcmp() strnstr()
memcpy() strdup()
memmove() toupper()
memchr() tolower()
bzero() atoi()
calloc()
// Additional functions
ft_substr() ft_strjoin()
ft_strtrim() ft_putnbr_fd()
ft_split() ft_putendl_fd()
ft_itoa() ft_putstr_fd()
ft_strmapi() ft_putchar_fd()
ft_striteri()
// Linked-list manipulation functions
ft_lstnew() ft_lstmap()
ft_lstadd_front() ft_lstiter()
ft_lstadd_back() ft_lstclear()
ft_lstsize() ft_lstdelone()
To see details of each function check the repository below, and the PDF with the instructions for the project, inside the repo: